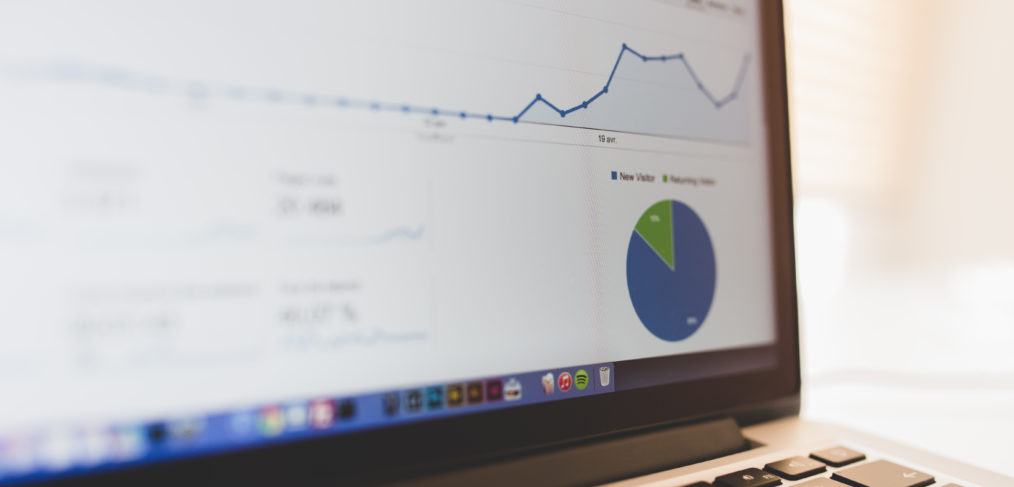
Passing clean meta tag data to Google Analytics
- What this does: Cleans text from meta tags using basic JavaScript to pass to Google Analytics reports
- Requirements: Google Analytics deployed through Tag Manager, understanding of regular expressions & JavaScript, admin access to Google Analytics
Over the years I’ve found one of the more useful tools in Google Analytics is the ability to pass custom data. Perhaps you want to build reports around blog tags, authors, or publish dates.
Each of these data can easily be passed to Google Analytics via custom dimensions if stored in meta tag fields.
At a high level it works like this:
- Create a custom JavaScript variable that returns the meta tag content
- Set up your custom dimension in Google Analytics
- Pass the custom JavaScript variable to the Google Analytics tag in Google Tag Manager
There already several great tutorials out there to explain this process. This tutorial explains how to capture meta and schema data and pass to Google Analytics. This tutorial explains how to capture open graph tag content.
Cleaning the text
However, you may run into a situation where the data needs some cleaning and you do not want to pass the text as is. For example, I was trying to capture data from a meta keywords tag recently, but the data looked like this:
<meta name="keywords" content=" , , , , News , , Sports, ">
As you can see, there were extra spaces and commas and I did not want to pass these to my Google Analytics report. But with a couple simple JavaScript string methods, we can send just data that looks like “News, Sports”
First, create a custom JavaScript variable in Tag Manager.
We’ll start by creating our function and check to see if the page even has a meta keywords tag. We’ll use an if else statement that will return the cleaned tags if they exists or an empty string if they do not exist.
This tutorial uses meta keywords, but you could easily replace “keywords” with another meta tag.
function myFunction() { var selection = document.querySelector('meta[name="keywords"]'); if (selection !== null) { } else { return ""; } }
Within the “if” statement, we’ll start manipulating the content of the meta keywords tag. First, we’ll create a variable that stores the value of the “content” attribute. Then we’ll eliminate the extra spaces after the commas.
var content = document.querySelector('meta[name="keywords"]').content; var reg = /, /g; var str1 = content.replaceAll(reg, ',');
This produces “,,,,News,,Sports,” Next we’ll consolidate places where there are multiple simultaneous commas to just one comma using the regex + modifer.
var reg2 = /,+/g; var str2 = str1.replaceAll(reg2, ',');
This produces “,News,Sports,” Next we’ll want to get rid of any extra commas at the beginning and end using regular expression anchors.
var reg3 = /^,/; var str3 = str2.replace(reg3, ''); var reg4 = /,$/; var str4 = str3.replace(reg4, '');
Almost done. We now have “News,Sports” The last step is put a space after any existing commas.
var reg5 = /,/g; var str5 = str4.replace(reg5, ', '); return str5;
That’s it! We know have “News, Sports” Here is the final function:
function myFunction() { var selection = document.querySelector('meta[name="keywords"]'); if (selection !== null) { var content = document.querySelector('meta[name="keywords"]').content; var reg = /, /g; var str1 = content.replaceAll(reg, ','); var reg2 = /,+/g; var str2 = str1.replaceAll(reg2, ','); var reg3 = /^,/; var str3 = str2.replace(reg3, ''); var reg4 = /,$/; var str4 = str3.replace(reg4, ''); var reg5 = /,/g; var str5 = str4.replace(reg5, ', '); return str5; } else { return ""; } }
Custom dimension
From here you would save your custom JavaScript variable.
Make sure you have a custom dimension set up in Google Analytics (Admin -> Custom Definitions -> Custom Dimensions).
Keep track of the index number of the custom dimension you just created. If it’s your first, it will be 1.
Now go back to Tag Manager and locate your Google Analytics Tag.
Check the box to “Enable overriding settings in this tag”
Under More settings, locate Custom dimensions. Place the matching index number in the Index column. In the Dimension Value field, find the custom JavaScript variable you just created.